Nested Loops
Python allows to use one loop within another just like the statements in a loop body.
The syntax for a nested while loop is :
while test-expression:
statement(s)
while test-expression :
statement(s)
Example
1 x=1
2 while x<=4: #Outer while loop
3 y=1 #body of outer loop begins
4 while y<=2: #inner (nested) loop
5 print(y) #body of inner loop begins
6 y+=1 #inner loop body ends
7 x+=1 #outer loop body ends
8 print("Out of Loops")
Working :
Line Program flow
1 Initializes x to 1
2 Outer loop, test expression is evaluated; x<4 if true control goes to line 3 else it goes to line 8
3 Initializes y to 1.
4 Inner loop, test expression is evaluated; y<2, if true control goes to line 5 else it goes to line 7
5 Prints value of y
6 Increments y by 1 (update expression). Control goes back to line 4
7 Increments value of x by 1 (update expression). Control goes back to line 2
For each value of outer loop, the inner loop iterates completely. Thus in the above example for each value of x, two values of y i.e. 1, 2 will be printed.
Output:
1
2
1
2
1
2
1
2
Similarly we can have a nested for loop. The syntax will be :
for variable in sequence:
statement(s)
for variable in sequence:
statement(s)
Example
for i in range(3)
for j in range(2)
print(i*j)
Workflow:
Output:
0
0
0
1
0
2
A for loop can nest a while loop and vice versa.
Example program :Program to print the average marks of 2 students in a class
Jump statements: break and continue
The jump statements forcibly alter the flow of control in a loop. This means they may force a loop to stop or skip an iteration as the case may be. There are two such statements in Python:
1. break
2. continue
The break statement
The break statement terminates the loop completely and the control goes to the line following the loop.
Example:
for i in range(1,7): # i iterates from 1 to 7
if i % 3 == 0:
break #loop terminates when number(i) is divisible by 3
print("Square is ", i ** 2) # prints the square of i
print("Loop Over")
Output:
Square is 1
Square is 4
Loop Over
Explanation :
The print statement is executed till the value of i is 2. When the if expression becomes true, the control comes out of the loop to the line after the loop.
Workflow
The figure below depicts the working of the above loop:
The continue statement
The continue statement simply skips the current iteration of the loop and control goes back to the top of the loop. This means that the code after continue statement is skipped.
Example:
for i in range(1,7): # i iterates from 1 to 7
if i % 3 == 0:
continue #loop skips an iteration when number(i) is divisible by 3
print("Square is ", i ** 2) # prints the square of i
print("Loop Over")
Output:
Square is 1
Square is 4
Square is 16
Square is 25
Loop Over
Explanation :
The print statement is executed for all values of i except 3. When the if expression becomes true, the control oes to the top and i is assigned the next value, i.e. 4.
Workflow:
The figure below demonstrates the difference between break and continue:
Using break and continue in nested loops
The break statement in a nested loop brings the control out of the immediate loop. The example below illustrates this.
i=1
while i<4:
x=1
while x<6:
if x%3==0:
break #when x becomes divisible by 3 the inner loop breaks, control goes to the outer loop
print (i,":",x)
x+=1
print("Outer") #when the inner loop breaks this statement of outer loop is executed
i+=1
Output:
1 : 1
1 : 2
Outer
2 : 1
2 : 2
Outer
3 : 1
3 : 2
Outer
Another example demonstrating the working of a break statement in outer loop.
i=1
while i<7:
if i%3==0: #Loop breaks when i becomes divisible by 3, control goes to statement after loops
break
x=1
while x<6:
print (i,":",x)
x+=1
print("Outer")
i+=1
print("Out of loops")
In the above example the outer loop iterates only till the value of i is 2. The inner loop iterates 5 times for each value of i.
Output:
1 : 1
1 : 2
1 : 3
1 : 4
1 : 5
Outer
2 : 1
2 : 2
2 : 3
2 : 4
2 : 5
Outer
Out of loops
The loop else statement
There is an optional else statement in Python loops.
The syntax is :
while test-expression:
statement(s)
else:
statement(s)
This else statement executes only when the loop terminates normally. This means it executes when a test expression in a while loop evaluates to false or when the for loop variable has completed all the iterations. It does not get executed when a loop is forced to terminate with a break statement.
Example 1:(Else is executed)
i=1
while i<8:
if i%6==0:
print ("I=", i)
i=i+1
else:
print("Else executed")
print ("Loop ends")
Output:
I= 6
Else executed
Loop ends
Example 2:(Else is Not executed)
i=1
while i<8:
if i%6==0:
break
print ("I=", i)
i=i+1
else:
print("Else executed")
print ("Loop ends")
Output:
I= 1
I= 2
I= 3
I= 4
I= 5
Loop ends
List Comprehension in Python
We can also use for loops to create lists in Python using list comprehension. The general syntax for creating lists in this way is :
listvariable=[ list element(iterator) for iterator in range(n)]
Example:
list2=[i for i in range(4) ]
print(list2)
Output:
[0, 1, 2, 3]
We can also use conditions while forming lists.
Example:
list2=[i for i in range(25) if i % 2==0]
print(list2)
Output:
[0, 2, 4, 6, 8, 10, 12, 14, 16, 18, 20, 22, 24]
Using Strings, we can create lists of alphabets.
Example:
list1=[i for i in 'humane']
print(list1)
Output:
['h', 'u', 'm', 'a', 'n', 'e']
Example:
str1=input("Enter a word :")
list1=[i for i in str1 if str1.isupper()]
print(list1)
Output:(option 1)
Enter a word :GOOD MORNING
['G', 'O', 'O', 'D', ' ', 'M', 'O', 'R', 'N', 'I', 'N', 'G']
Output:(option 2)
Enter a wordGood Morning
[]
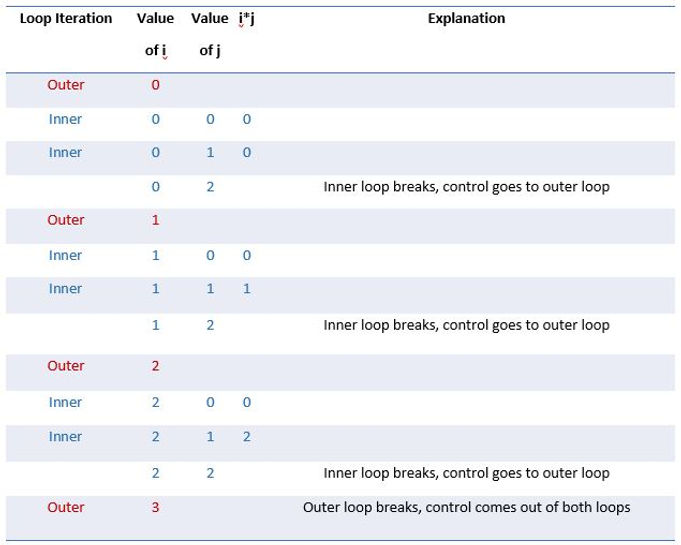

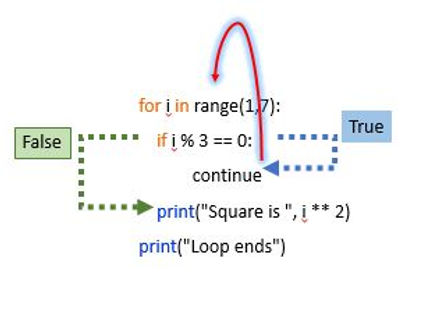
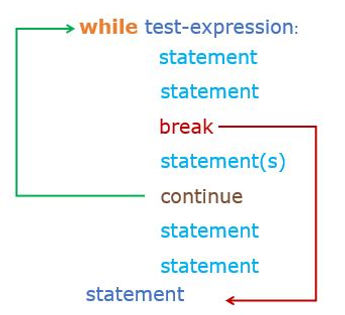