Python Dictionaries
Table of Contents
Dictionaries: Introduction
Defining a Dictionary
Creating a Dictionary
Accessing Dictionaries
Adding and updating elements in a Dictionary
Operators and built in Dictionary Methods
-
in and not in operator
-
dict.len()
-
dict.items()
-
dict.values()
-
dict.keys()
-
dict.get(key)
-
dict.update()
-
dict.clear()
-
dict.pop(key)
-
dict.popitem()
-
del
-
dict.fromkeys()
-
dict.copy()
-
dict.setdefault()
-
max()
-
min()
-
sorted()
Sample Programs
Introduction
Dictionaries are a way of representing arrays with keys in Python. It can be considered as an unordered list of items with each element referenced by a key.
Features of Dictionaries:
1. They are mutable, ie the values may change , although the keys cannot be changed.
2. They may grow or shrink, ie elements can be added or deleted as required.
3. The elements are referenced by a key.
4. Each key in a dictionary has to be unique.
We can say that a dictionary is an unordered list of items where every item is a key-value pair.
Defining a dictionary
We can define a Python dictionary using a series of key-value pairs enclosed in curly brackets.
>>> D={1:"Ankit", 2:"Babita", 3:"Chirag", 4:"Dorothy"}
>>> D
{1: 'Ankit', 2: 'Babita', 3: 'Chirag', 4: 'Dorothy'}
Representation of D:
A dictionary can be created in a number of ways:
1. By directly assigning comma separated key-value pairs enclosed in a pair of curly braces to a valid identifier.
The statement below creates a dictionary d1, having four elements all of the same type.
>>>d1={'Country':'Australia', 'Capital':'Canberra', 'Area':'7,682,300 sq. km', 'Climate':'Temperate'}
The following statement creates a dictionary having 4 fractional values with keys from 1 to 4.
>>> d={1:3.14, 2:2.67, 3:4.88, 4:6.90}
>>> d
{1: 3.14, 2: 2.67, 3: 4.88, 4: 6.9}
In the statement below we can see that the dictionary is created with 6 elements with two values of type string.
>>> d={1:3.14, 2:2.67, 3:4.88, 4:6.90, 5:"Hearty Brown", 6:"5 ft 6 in"}
>>> d
{1: 3.14, 2: 2.67, 3: 4.88, 4: 6.9, 5: 'Hearty Brown', 6: '5 ft 6 in'}
Dictionary with keys of different data types:
>>> d={1:"Forenoon", "Time":13.25}
>>> d
{1: 'Forenoon', 'Time': 13.25}
2.Using the function dict(),we can create an empty dictionary.
>>>d=dict()
>>>d
{}
We can create an empty dictionary by assigning a pair of empty curly brackets to an identifier.
>>>d={}
>>>d
{}
We can now add elements to the dictionary using square brackets :
>>> d={}
>>> d[1]="Rat"
>>> d[2]="Bat"
>>> d[3]="Mat"
>>> d[4]="Cat"
>>> d
{1: 'Rat', 2: 'Bat', 3: 'Mat', 4: 'Cat'}
Note: Python automatically assigns the keys to the values.
Sample Program :
Write a Python script to generate and print a dictionary that contains a number as the key and its square as the value in the form(x:x*x). The dictionary should contain n elements where n is input by the user.
Example if n = 4, then the dictionary should look like :
{1: 1, 2: 4, 3: 9, 4: 16}
Accessing dictionaries
1. To access the individual elements, we can use the key value enclosed in square brackets.
>>> print(d[1])
Rat
2. To access the whole dictionary i. e. to traverse a dictionary, we can use a loop.
>>> for i in d:
print(i)
1
2
3
4
#Prints the keys of the dictionary corresponding to the value of i (loop iterator).
>>> for i in d:
print(d[i])
Rat
Bat
Mat
Cat
#Prints the values of the dictionary corresponding to the keys represented by value of d[i].
>>> for i in d:
print(i,":",d[i])
1 : Rat
2 : Bat
3 : Mat
4 : Cat
#Prints the key-value pairs of the dictionary
We can also use some built in functions with loops to access the elements of a dictionary, a. We shall cover this a bit later on this page.
Adding and Updating elements in a dictionary
1. Adding elements: We can add elements to a dictionary, ie key-value pair using simple assignment.
>>> d={1:"Amrit", 2:"Bhavesh", 3:"Chetan", 4:"Dinesh"}
>>> d[5]="Kartikay"
>>> d
{1: 'Amrit', 2: 'Bhavesh', 3: 'Chetan', 4: 'Dinesh', 5: 'Kartikay'}
>>> d['Stream']='Science'
>>> d
{1: 'Amrit', 2: 'Bhavesh', 3: 'Chetan', 4: 'Dinesh', 5: 'Kartikay', 'Stream': 'Science'}
2. Updating elements: We can update existing elements in a dictionary by simple using the assignment operator. For example to change the value of the element referenced by the key 4 to 'Falguni', we can write the following command:
>>> d[4]='Falguni'
>>> d
{1: 'Amrit', 2: 'Bhavesh', 3: 'Chetan', 4: 'Falguni', 5: 'Kartikay'}
Note: To change or update an element in a dictionary, we can assign a value to an existing key. But if the key is not present in the dictionary, a new element , the key-value pair is added to the dictionary.
The following statement adds an element to the dictionary:
>>> d[6]='Prerna'
>>> d
{1: 'Amrit', 2: 'Bhavesh', 3: 'Chetan', 4: 'Falguni', 5: 'Kartikay', 6: 'Prerna'}
Sample program:
WAP to add records to a dictionary 'fruits' .
Operators and built in Dictionary methods
1. in and not in: These membership operators check whether a given element belongs to the dictionary or not, respectively.
Example :
>>>d={1: 'Amrit', 2: 'Bhavesh', 3: 'Chetan', 4: 'Falguni', 5: 'Kartikay', 6: 'Prerna'}
>>> 2 in d
True
When we use in or not in to check for the presence of a value with dictionary name , the key is checked. The value only is not checked and we get the answer as false even if it is present in the dictionary.
Example:
>>> 'Prerna' in d
False #No key
To check for a value in the dictionary, we need to use the values() function.
Example:
>>> 'Prerna' in d.values()
True
The values are case sensitive.
Example:
>>> 'prerna' not in d #case sensitivity
True
2. len()
This function returns the number of elements i.e. the key-value pairs present in the dictionary.
d={1: 'Amrit', 2: 'Bhavesh', 3: 'Chetan', 4: 'Falguni', 5: 'Kartikay', 6: 'Prerna'}
Example:(considering the above dictionary, d)
>>> len(d)
6
3. d.items()
This function returns the list of elements in the dictionary.
>>>d={1: 'Amrit', 2: 'Bhavesh', 3: 'Chetan', 4: 'Falguni', 5: 'Kartikay', 6: 'Prerna'}
>>> d.items()
dict_items([(1, 'Amrit'), (2, 'Bhavesh'), (3, 'Chetan'), (4, 'Falguni'), (5, 'Kartikay'), (6, 'Prerna')])
To view the items as a list we can write the following statement:
>>> list(d.items())
[(1, 'Amrit'), (2, 'Bhavesh'), (3, 'Chetan'), (4, 'Falguni'), (5, 'Kartikay'), (6, 'Prerna')]
4. d.keys()
This function returns the all the keys that are present in the dictionary.
Example:
>>> d.keys()
dict_keys([1, 2, 3, 4, 5, 6])
5. d.values()
This returns all the values in the dictionary.
Example:
>>> d.values()
dict_values(['Amrit', 'Bhavesh', 'Chetan', 'Falguni', 'Kartikay', 'Prerna'])
6. d.get(key)
This function returns the value corresponding to a key in the dictionary. If the key is not present, the function returns, 'None'.
>>> d.get(2)
'Bhavesh' #It returns the name 'Bhavesh' corresponding to the key, 2.
>>>d.get(8) #It returns the none as key 8 is not present in d.
None
We can also specify a default value to be displayed in case the key is not present in the dictionary.
>>> d.get(8, -1)
-1
In the above example, the default value is set to -1, which means if the key is not present in the given dictionary, then the command returns -1.
7. d.update(object)
Here object can be a dictionary or an iterable with a key-value pair
This function merges the object enclosed in brackets with d.
Example:
>>>d={1: 'Amrit', 2: 'Bhavesh', 3: 'Chetan', 4: 'Falguni', 5: 'Kartikay', 6: 'Prerna'}
>>> d2={7:"Lara", 8:"Lori"} # adding key- value pairs
>>> d.update(d2)
>>> d
{1: 'Amrit', 2: 'Bhavesh', 3: 'Chetan', 4: 'Falguni', 5: 'Kartikay', 6: 'Prerna', 7: 'Lara', 8: 'Lori'}
Note: For each key in the object:
i. If the key is present in the dictionary, d, the value is updated.
ii. If the key is not present, then a new key-value pair is added to the dictionary.
Example:
>>> d4={2:"Jayesh", 9:"Sameeksha"}
>>> d.update(d4) #adding a new dictionary
>>> d
{1: 'Amrit', 2: 'Jayesh', 3: 'Chetan', 4: 'Falguni', 5: 'Kartikay', 6: 'Prerna', 7: 'Lara', 8: 'Lori', 9: 'Sameeksha'}
Here since the key 2 already exists in the dictionary, the name is changed to Jayesh. Also the pair 9:"Sameeksha" is added since it does not exist in the dictionary.
8. d.clear()
This function clears the entire dictionary. It deletes all the key-value pairs.
>>>d2={1:"Deer", 2:"Bear", 3:"Cat", 4:"Elephant"}
>>>d2
{1:"Deer", 2:"Bear", 3:"Cat", 4:"Elephant"}
>>>d2.clear()
>>>d2
{}
9. d.pop(key)
This function removes a key along with its value in a dictionary.
>>> d2={1:"Apple", 2:"Ball", 3:"Pineapple",4:"Mangoes"}
>>> d2
{1: 'Apple', 2: 'Ball', 3: 'Pineapple', 4: 'Mangoes'}
>>> d2.pop(2)
'Ball'
>>> d2
{1: 'Apple', 3: 'Pineapple', 4: 'Mangoes'}
>>> d2.pop(1)
'Apple'
>>> d2
{3: 'Pineapple', 4: 'Mangoes'}
If we try to remove a non existent key from the dictionary, an exception is generated.
>>> d2.pop(6)
Traceback (most recent call last):
File "<pyshell#7>", line 1, in <module>
d2.pop(6)
KeyError: 6
We can provide with a default value which will be displayed if the key we pop is not in the dictionary.
>>> d2.pop(6,-1)
-1
Thus the value -1 will be returned if we try to pop a non existent key.
10. popitem()
This function removes the last element inserted from a dictionary. It also returns the removed key value pair.
Example:
d2={1:"Bat",2:"Cat",3:"Rat",4:"Mat"}
print(d2)
d2.popitem() #the last key value pair i.e. 4:"Mat" is removed
print(d2)
Output:
{1: 'Bat', 2: 'Cat', 3: 'Rat', 4: 'Mat'}
{1: 'Bat', 2: 'Cat', 3: 'Rat'}
The elements are deleted in a LIFO (Last In First Out) manner i.e, the element which is inserted first is deleted first.
11. del
This(del) is actualy statement that can be used to remove a key value pair from a dictionary.
Example:
d={1:"GATE",2:"GRE", 3:"CAT", 4:"ASL"}
print(d)
del d[2] # To delete key value 2
print(d)
Output:
{1: 'GATE', 2: 'GRE', 3: 'CAT', 4: 'ASL'} # original Dictionary
{1: 'GATE', 3: 'CAT', 4: 'ASL'} # dictionary after removing key value 2
The function will generate an error if we try to use it with a non existent key.
Example:
d={1:"GATE",2:"GRE", 3:"CAT", 4:"ASL"}
del d[6]
print(d)
Output:
Traceback (most recent call last):
File "C:/Users/Neeru/AppData/Local/Programs/Python/Python38-32/d1.py", line 3, in <module>
del d[6]
KeyError: 6
12. fromkeys(sequence , value)
where:
sequence is a list of values which represent the keys
value parameter provides the value to be given to all the keys. It can be single value or a list of values..
The fromkeys() method creates a new dictionary from a given sequence of elements and values. The sequence can be a tuple or list containing values. these form the keys of the dictionary. The value parameter is optional but if provided assigns the given value to the keys obtained from the sequence.
Example:
x=(1,2,3,4)
y=20
d1=dict.fromkeys(x,y)
print(d1)
Output:
{1: 20, 2: 20, 3: 20, 4: 20}
in the above example the keys are formed from the iterable x and all the keys are assigned the value given by 20. We can also assign a list of values to the keys.
Example:
x=[1,2,3,4]
y=(20,25,60,65)
d1=dict.fromkeys(x,y)
print(d1)
Output:
{1: (20, 25, 60, 65), 2: (20, 25, 60, 65), 3: (20, 25, 60, 65), 4: (20, 25, 60, 65)}
13. copy()
The copy() function creates a shallow copy of an existing dictionary. This means that the original dictionary does not get changed if we make changes to the copied one.
Example:
d1={1:"Bat",2:"Cat",3:"Rat",4:"Mat"}
d2=d1.copy()
print(d2)
print(d1)
Output:
{1: 'Bat', 2: 'Cat', 3: 'Rat', 4: 'Mat'} #d2 is a copy of d1
{1: 'Bat', 2: 'Cat', 3: 'Rat', 4: 'Mat'} #d1 is printed
Note: Making changes to d2 will not change d1
Example:
d1={1:"Bat",2:"Cat",3:"Rat",4:"Mat"}
d2=d1.copy()
d2.pop(2) # removing key value 2
print(d2)
print(d1)
Output:
{1: 'Bat', 3: 'Rat', 4: 'Mat'}
{1: 'Bat', 2: 'Cat', 3: 'Rat', 4: 'Mat'} # no change to d1
We can also use the '=' operator to make a copy of a dictionary.
Example:
d1={'Name':"Victoria", 'Age':65, 'City':"London", 'MStatus':"Married"}
d2=d1
print(d2)
Output:
{'Name': 'Victoria', 'Age': 65, 'City': 'London', 'MStatus': 'Married'}
Difference between using = and copy()
The = operator creates a deep copy of the dictionary. this means that changes made to the copy are reflected back to the original dictionary.
Example:
d1={'Name':"Victoria", 'Age':65, 'City':"London", 'MStatus':"Married"}
d2=d1
print(d2)
d2.pop("MStatus") #removing a key value pair
print(d1) # printing original
Output:
{'Name': 'Victoria', 'Age': 65, 'City': 'London', 'MStatus': 'Married'} #copied Dictionary
{'Name': 'Victoria', 'Age': 65, 'City': 'London'} # original Dictionary after changes
14. setdefault()
The method setdefault() is used to return the value of a key which exists in the dictionary. If the key does not exist then the setdefault() function inserts the key with the given value.
When the key is present in the dictionary:
Example:
furniture = {"Name": "Chair", "Model": "Rocking Chair", "Material": "Wood"}
x = furniture.setdefault("Model", "Rocking Chair")
print(x)
print(furniture)
Output:
Rocking Chair
{'Name': 'Chair', 'Model': 'Rocking Chair', 'Material': 'Wood'}
When the key is not present in the dictionary:
Example:
furniture = {"Name": "Chair", "Model": "Rocking Chair", "Material": "Wood"}
x = furniture.setdefault("color", "Dark Brown")
print(x)
print(furniture)
Output:
Dark Brown
{'Name': 'Chair', 'Model': 'Rocking Chair', 'Material': 'Wood', 'color': 'Dark Brown'} #the key-value is added
15. max()
The max() function is used to find the key with the maximum value in a dictionary. It uses the key get function.
Example:
IMDb = {'The Umbrella Academy' : 8.0,'Friends':9.8, 'Stranger Things':8.8, 'Russian Doll':8.0}
highest = max(IMDb, key=IMDb.get) #get retrieves the key with the highest value
print(highest)
Output:
Friends
16. min()
The min function retrieves the key with the lowest value in a dictionary.
Example:
IMDb = {'The Umbrella Academy' : 8.0,'Friends':9.8, 'Stranger Things':8.8, "Russian Doll":8.0}
lowest = min(IMDb, key=IMDb. get)
print(lowest)
Output:
The Umbrella Academy
NOTE: If we have more than 1 key with lowest(or highest) value in a dictionary then the value first occurrence is printed.
17. sorted()
The sorted() function can be used to sort a dictionary by value ,by key or by key value pair using the various in built dictionary methods.
Sorting by key
When applied using the dictionary keys() function the sorted() method returns the data in a dictionary in ascending order of the keys. The values of corresponding to the keys are not changed.
Example:
d1={4:"Apple", 1:"Oranges",3:"Plum", 2:"Grapes"}
print(sorted(d1.keys()))
Output:
[1, 2, 3, 4]
Sorting by value
The sorted() function when used with the values() method of dictionary returns the values in a dictionary in ascending order.
Example:
d1={4:"Apple", 1:"Oranges",3:"Plum", 2:"Grapes"}
print(sorted(d1.values()))
Output:
['Apple', 'Grapes', 'Oranges', 'Plum']
Sorting by key-value pair
The sorted function can be used in combination with the items method of dictionary to return the key-value pair in a sorted order in the dictionary.
Example:
d1={4:"Apple", 1:"Oranges",3:"Plum", 2:"Grapes"}
print(sorted(d1.items()))
Output:
[(1, 'Oranges'), (2, 'Grapes'), (3, 'Plum'), (4, 'Apple')]
In all the above scenarios the sorted function returns the values of the dictionary in a sorted manner . However the dictionary is not sorted.
Example:
d1={4:"Apple", 1:"Oranges",3:"Plum", 2:"Grapes"}
print(sorted(d1.items()))
print(d1)
Output:
[(1, 'Oranges'), (2, 'Grapes'), (3, 'Plum'), (4, 'Apple')] # sorted values are returned and printed
{4: 'Apple', 1: 'Oranges', 3: 'Plum', 2: 'Grapes'} # original dictionary is not changed
We can use a second dictionary to store the sorted values.
Example:
d1={4:"Apple", 1:"Oranges",3:"Plum", 2:"Grapes"}
d2=sorted(d1.items()) # storing the sorted value in the second dictionary print(d2)
Output:
[(1, 'Oranges'), (2, 'Grapes'), (3, 'Plum'), (4, 'Apple')]
Sorting a dictionary in descending order
We can also sort a dictionary in descending order using the revers parameter.
Example:
d1={4:"Apple", 1:"Oranges",3:"Plum", 2:"Grapes"}
print(sorted(d1.items(), reverse=True))
Output:
[(4, 'Apple'), (3, 'Plum'), (2, 'Grapes'), (1, 'Oranges')]
Sample Program:
Write a Python script to search for a given value in a Dictionary.
Sample Program:
WAP to count the number of elements in a dictionary.
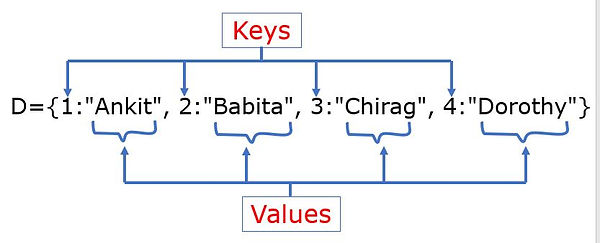