पाइथन शब्दकोश
Modular Programming
Modular programming refers to the breaking of large programs into smaller manageable pieces of code or modules. They are referred to by user defined names.
Python Modules consist of lines of Python code that perform a specific task. They help in modularizing programs. Modules can be of three types in Python:
1. Inbuilt modules
2. Functions (Inbuilt and User Defined)
3. Packages
In this chapter we shall be covering Python inbuilt modules. Python offers numerous modules that contain functions which perform specific tasks. We can also say that a group of functions that perform specific category of tasks are grouped together to form a Python module. For example the math module contains functions that perform mathematical calculations.
In the context of this chapter we shall be covering three main modules viz math, random and statistics module.
Using a Module
We can use the import statement in three ways to be able to use functions from a particular module.
1. import <module-name>
For example, in order to find the square root of a given number, we can use the sqrt() function from the math module, we can write the following code:
import math
x=int(input("Enter a number"))
print(math.sqrt(x))
Output:
Enter a number 81
9.0
We can also use the following two ways to import modules and use the functions they contain:
2. from <module-name> import <method-name>
Example:
from math import sqrt
print(sqrt(81))
Output:
9.0
3. from <module-name> import *
Example:
from math import *
print(sqrt(81))
Output:
9.0
Note that in the first method we need to specify the module name followed by the function name in order to use the function.
Math module
The math module contains functions that can be used to perform mathematical tasks. Given below is the list of functions i the math module.
Random module
This module contains functions to generate random numbers between two values. Among other uses the random module can be used to display elements randomly from a list , dictionary etc.
Using the random module
1. random()
This function generates a random number between 0.0 and 1.0(not including) . It des not accept any argument in the brackets.
Example:
import random
print(random.random())
Output:
0.7489153508852794
In order to get a larger number or use it as part of a larger calculation, we can multiply it by an integer.
Example -To generate a number between 1.0 and 10.0:
import random
print(random.random()*10)
Output:
7.168087410165594
2. randitn(x,y)
The randint() function accepts two arguments and generates a random number between the two. For example if the arguments are x and y it will generate an integer value between x and y.
Example:
import random
print(random.randint(2,7)) #will generate a number between 2 and 7, inclusive
Output:
2
We can also use the randint() function to generate and assign a random number to a variable. For example; p=randint(9,11), will assign a value between 9 and 11 to the variable p.
The randint() function can be implemented in numerous ways.
Example:
Consider the code below:
import random
y=random.randint(4,9) #y will be assigned a value between 4 and 9
for i in range(1,y+1): # depending on the value of y, the loop will run from 1 to n where 4<=n<=9
print(i, end=" ")
In the above code y can have value between 4 and 9. Thus the loop will run from 1 to n, n being in the range 4-9. Thus we can have any one of the following outputs in this case:
option 1: 1 2 3 4
option 2: 1 2 3 4 5
option 3: 1 2 3 4 5 6
option 4: 1 2 3 4 5 6 7
option 5: 1 2 3 4 5 6 7 8
option 6: 1 2 3 4 5 6 7 8 9
Here the minimum value generated for y is 4 and maximum 9.
Let's take a look at another example:
import random
x=random.randint(2,4)
y=random.randint(4,7)
for i in range(x,y+1):
print(i, end=" ")
There are two random numbers generated in the above code, x and y. The minimum and maximum values that the variables x and y will assume are as follows:
| x | y
_________________
min | 2 | 4
_________________
max | 4 | 7
Thus the loop will give output starting with anything between 2 - 4 and ending between 4-7.
3. randrange (x,y,z)
This function also generates a random integer between x and y-1. There is also a step value, z which has a default value of 1. Also the step parameter is optional.
Example:
import random
y=random. randrange (4,21,2) # y will be assigned value between 4 and 20 which are even as step is 2. So it can be
#4,6,8,10,12,14,16,18,20
print(y)
Output:
18
In the above example y will be assigned a value between 4 and 20 in multiples of 2.
Statistics Module
This module offers functions that perform statistical tasks on numeric data. There are numerous functions in this module. We shall cover three of these, viz, mean(), median( )and mode().
1. mean()
This function will return the average of a list of values.
Example:
import statistics
list1=[3,4,20,7,9,8]
print(statistics.mean(list1))
Output:
8.5
In the above code the numbers in the list are are added and then divided by 6 to give average or mean.
2. median()
The function median() returns the middle value in a list of given numbers/data by first arranging them in ascending order and then finding the exact middle element.
Example:
import statistics
list1=[3,4, 7,9,8] #when ordered, the list will be; 3,4,7,8,9 and the middle is 7
print(statistics.median(list1))
Output:
7
If the list contains even numbers then the two middle numbers (after arranging the list) are added and divided by two to get the median.
Example:
import statistics
list1=[2,15,4,6,8,9] #ordered list will be : 2,4,6,8,9,15 and the middle two values are 6,8. So median=(6+8)/2=7
print(statistics.median(list1))
Output:
7.0
3. mode()
This function returns the value in a given list that occurs the most number of times.
Example:
import statistics
list1=[2,15,4,6,8,9,4,9,8,4,9,9,7]
print(statistics.mode(list1))
Output:
9
NOTE: If there are more than one values in the list having same number of occurrences, then this function returns the first number.
Example:
import statistics
list1=[2,15,4,6,8,9,4,9,8,4,9,9,7,4] # 4 and 9 each occur four times in the list
print(statistics.mode(list1))
Output:
4
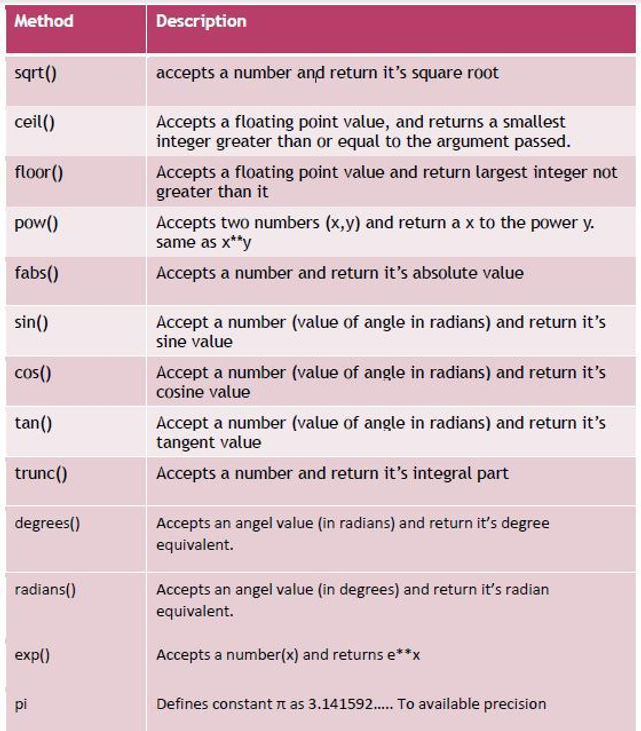
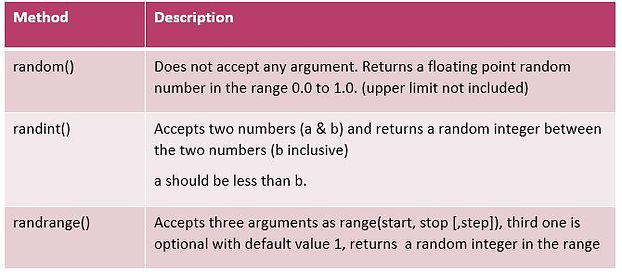
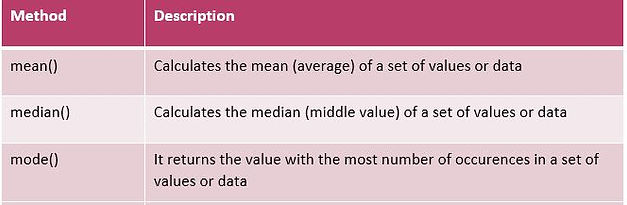