Variables and Data Types
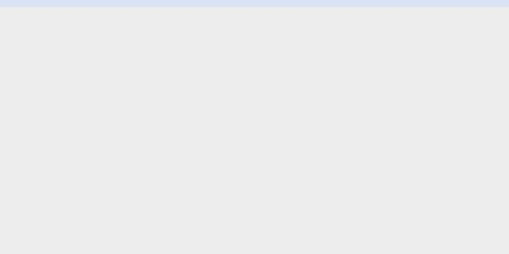

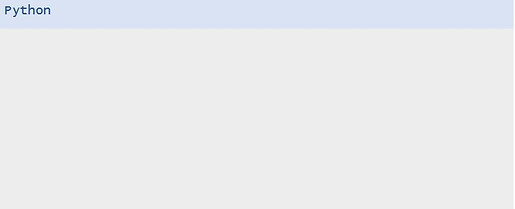



The L-value must be a variable and the R-value must be a constant of a valid type. We can not have a constant on the LHS.
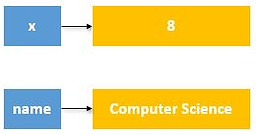

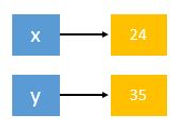

x is first assigned the value 45, so the data type is int
x is now assigned the value "good Morning". The data type automatically changes to str
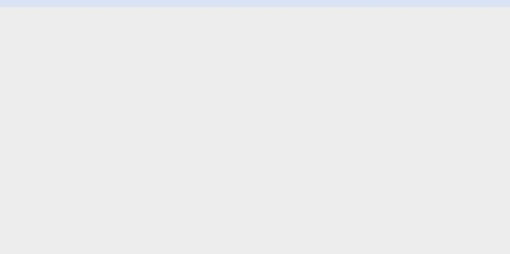


Variables
In Python, variables are treated as objects. This means that a variable in Python refers to a value stored in the computer memory.
Every variable has a:name, value and a data type associated with it. So the statement :
>>>x = 8
declares a variable with the name x , having value 8 and type integer .
Let us consider another example :
>>>name="Computer Science"
In the above statement a variable name of the type string is declared with the value "Computer Science".
So the general statement of declaring a variable with a value will be:
variable name(identifier) = value/constant
(L-value) (R-value)
The variable will be assigned the value given on the right hand side.
Example:
>>> a=90
>>> a
90
In the above code the value 90(R-Value) is assigned to variable a(L-Value)
>>> 56=b
SyntaxError: can't assign to literal
The above statement generates an error because L-value is a constant.
Identifiers
The name of a variable is also called an identifier. An identifier is a name given to any object in Python. When we name an object some rules have to be followed. These are :
-
The first character of the identifier must be a letter of the alphabet (uppercase or lowercase character ) or an underscore (_).
-
The rest of the identifier name can consist of letters (uppercase or lowercase ), underscores (_) or digits (0-9).
-
Python is a case sensitive language. Thus identifier names are also case-sensitive. For example, RollNo and rollno are not the same.
-
Examples of valid identifier names are _age, name, a9999, Student_Id.
-
A keyword cannot be used as an identifier
-
Examples of invalid identifier names are .
Invalid identifier Reason
Admit card space
45lane starting with digit
All*gotit special character
d+f + not allowed
while keyword
Data Types
A data type defines a set of values and the operations that can be performed on an object/variable of that type...
Need for Data Types :
-
When programming, we store the data in our computer's memory. As we all know that a computer is just a machine. It cannot by itself distinguish between various types of Data. This means that it cannot distinguish between the number 20 and the letter ‘A’ or the word “good” . For the computer all of this is just a piece of data.
-
It is the programmer who must tell the computer that 20 is a number, ‘A’ is a character and ‘good’ is a word. How do we do it?
-
By using data types we can classify the different data for the computer so that it can be stored and processed in a certain manner.
-
This is because all the data does not occupy the same amount of memory and they are not going to be interpreted the same way.
Python supports a large variety of data types.
The basic types are numbers, strings and boolean . We shall discuss the basic types here .
1. Numbers : These type of data include all numbers. All operations that can be performed on numeric data apply to these types. There are three sub types; integer, float and complex.
a. Integer : Whole numbers are treated as integers in Python. To declare a variable of type integer we can simply assign a whole number to a variable.
>>>x=45
Here x is a variable pointing to the constant 45.
In Python, an integer can be of any length. This means you can assign any whole number to an integer. It depends on the available memory. Typical examples to use integer variables would be to store age, roll number etc.
b. Floating point : Fractional numbers are treated as float type in Python. So for example 22.5, 45.69034 etc. are data of type float. Floating point variables can be used to store values such as salary, rate of interest etc.
>>>y=24.9008
Here the variable y is of type float. The float variables have a decimal point.
c. Complex : Python provides us with a way to represent complex numbers. Complex numbers have a real part and an imaginary part. For example A+Bj is a complex number where A is the real part and B is imaginary. Both A and B are floating point number and j represents the square root of an imaginary number.
Examples of complex numbers:
x=4+7j
y=2-6j
To extract the real and imaginary parts of a complex number z, we can use the statements, z.real, z.imag.
Example
>>>x=4+7j
>>>print(x.real, x.imag)
4.0 7.0
2. Strings. A string is a sequence of characters that can be taken from the Python character set.In Python strings are known as str. A string can include alphabets, numbers and special symbols enclosed in single ,double or triple quotes.
Examples of string declarations
>>>a='Good Morning'
>>>print(a)
Good Morning
>>>str1="Python#3.0 is a fun language"
>>print(str1)
Python#3.0 is a fun language
We can use single , double or triple quotes with strings in Python.
Using triple Quotes
Remember the single or double quotes in a string literal are delimiters, they are not a part of the string.
When we want to use single or double quotes in the string, we can use triple quotes.
>>> x=''' This is the girl's basketball team'''
>>> print(x)
This is the girl's basketball team
>>> print (''' She exclaimed "What an incredible book" ''')
She exclaimed "What an incredible book"
>>>
Also we can write multi line strings using triple quotes:
>>> x='''we are the best
people in
the world
so let us go!!!'''
>>> x
'we are the best\npeople in\nthe world\nso let us go!!!'
>>>
3. None : The none type in Python defines null or no value at all. It is different from the value 0. None is also a keyword in Python.To define a variable of none type, we write the following code :
>>> x= None
>>> print(x)
None
>>>y=None
>>>y
>>>
No value is printed when we type y at the prompt. Note the capital N in the keyword None.
4. Boolean : A variable of type Boolean can have any of the two values; true or false. We shall cover more on Boolean variables in the topic, control structures.
The type() method
Python has an inbuilt method or function called type which can be used to find out the type of variable at run-time. The syntax is :
type(constant/ variable name)
Using type with constant values:
>>> type(15)
<class 'int'>
>>> type(24.5)
<class 'float'>
>>> type("Python Programming")
<class 'str'>
>>> type(True)
<class 'bool'>
>>> type(None)
<class 'NoneType'>
Using type with variables:
>>> x=34
>>> type(x)
<class 'int'>
>>> y=45.99
>>> type(y)
<class 'float'>
>>> num=4+7j
>>> type(num)
<class 'complex'>
>>> z=None
>>> type(z)
<class 'NoneType'>
>>> city="Johannesburg"
>>> type(city)
<class 'str'>
Variables and Types
Python does not require us to explicitly declare the data type of a variable. The variable assumes the data type according to the value assigned to it. For example:
>>> x=34
>>> type(x)
<class 'int'>
34 is an integer or whole number, so x is of type int.
Similarly
>>>name="Casablanca"
>>>type(name)
<class 'str'>
Here the variable name is of type str.
The value in a variable can also vary. This means a variable can change its value in a program. For example:
>>>x=9
>>>print(x)
9
>>>x=34
>>>print(x)
34
Changing variable values and Dynamic Typing
In Python a variable is not a container of the value, rather a simple reference to a value stored in the memory.
The constant values are stored in the memory having a fixed address. A variable when assigned a constant value, points to that particular value.
Consider the given code and the corresponding memory map.
>>> x=24
>>> y=35
>>> x
24
>>> y
35
Now if we change the value of y :
>>>y=x
>>>y
24
x and y both have the value 24.
Now consider the following code :.
>>> x=9
>>> type(x)
<class 'int'>
>>> x=9.0
>>> type(x)
<class 'float'>
We can see that the data type of x is int at first and then it changes to float. This is unlike most of the programming languages where the data type of a variable remains static(cannot be changed), once declared.
In Python we can assign a variable with data of any type during the course of a program.The data type of a variable varies according to the value assigned to it. For example
>>>x=45
>>>x
45
>>>type(x)
<class 'int'>
>>>x="Good Morning"
>>>x
'Good Morning'
>>>type(x)
<class 'str'>
In the above code the data type of the variable x automatically changes when we assign a string literal to it. We can assign a value of any data type to a variable in the course of a code. This is known as Dynamic Binding.
Variables and assignments
A variable can be assigned a value.using the '=' (assignment operator).
1. We can assign the same value to multiple variables in the same statement.
variable1=variable2=variable3=.......=value
The statement above assigns the same value to variable1, variable2 etc.
Example:
tsum=count=0
2. We can also assign multiple values to multiple variables using a single statement:
var1,var2,var3,.....,valn=val1,val2,val3,.......,valn
Example:
name, Class, section= "Kartik", 12,"A"
The above statement will assign "Kartik" to name, 12 to Class and "A" to section.
Sample code:
>>>x=y=z=24
>>>x,y,z
>>>(24,24,24)
>>> a,b,c="Hello","How","are"
>>> a,b,c
('Hello', 'How', 'are')
>>> a,b,c="hello",2,9.8
>>> a,b,c
('hello', 2, 9.8)
Type Conversions
Python provides some simple built in functions to convert variables from one type to the other:
1. int()
Converts an object to integer. The object can be a float variable or a string made up of numbers.
Example:
>>> x=9.0 # Here x is a float variable
>>> print(int(x))
9
>>> y="99" #Here x is a string
>>> print(int(y))
99
2. float()
Converts an object to float. The object can be an integer variable or a string made up of decimal numbers.
Example:
>>> x=45 # Here x is an int variable
>>> print(float(x))
45.0
>>> y="99.45" #Here y is a string
>>> print(float(y))
99.45
3. str()
Converts an object to a string type where the object n]can be an integer or float.
Example:
>>> q=38 #q is an integer
>>> str(q)
'38'
>>> p=90.99 #p is a float
>>> str(p)
'90.99'
4. chr()
This function gives the character representation if a number according to the ASCII code. (code which represents each character on the keyboard)
>>> z=90
>>>chr(z)
'Z'
This means Z (capital) is represented by the ASCII code 90.
Type casting
We can modify the data type of the result of an expression involving same or multiple data types. This is known as type casting.
Example 1:
>>> print(24.5+67.8)
92.3 #without typecasting
>>> print(int(24.5+67.8))
92 #casting to int()
Example 2:
>>> print(90+20)
110
>>> print(float(90+20)) #casting to float()
110.0
However, it is important to note that type casting just casts the value of an expression or variable, it does not change the original data type of a variable.
Example 3:
>>> x='90'
>>> print(int(x))
90
>>> x+80
Traceback (most recent call last):
File "<pyshell#6>", line 1, in <module>
x+80
TypeError: must be str, not int
In the above example, the variable x is of string type. We can cast it to int and display the value as int. But when we try to add it to an integer a type mismatch error is shown.
But we can add the cast value of x as shown in the example below
Example 4:
>>> print(int(x)+70) #casting x to int and adding in an expression
160
>>> b=45
>>> print(str(b))
45
>>> b+90
135