The order in which a set of statements are executed in a program is known as its flow of control
Generally the flow of control is sequential, ie., the statements are executed one after the other in their physical order of appearance. In sequential flow all the statements in the program are executed.
The programs or code we have seen till now are sequential.
However at times it is required to change this sequential flow so that only a particular statement or group of statements are executed. This is achieved by using control structures .
Control structures are statements that upon execution control the sequence of execution of statements in a program.
These can be categorized as follows:
-
Selection control statements
-
Iterative statements
Selection control statements
Selection control statements are used to make one times decisions in programs. They help choose a certain course of action depending on a given condition.
The if statement
This is the most basic type of control statement that can be used to implement decision making. The if statement is used to execute an instruction or a set of instructions only if a condition/Test expression is fulfilled or satisfied. The syntax is :
if test expression:
statement
In the above Syntax :
-
if is a keyword
-
Test expression is a valid relational / logical conditional expression which evaluates to true or false
-
statement is a valid Python executable statement. There can be more than one statement
The statement is executed only if the test expression evaluates to true.Note the colon after expression. It is part of the syntax and is mandatory. Also note the indentation in the second line. This indicates the body of if . If more than one statement needs to be executed , all of them must start with this indentation. The first unindented line of code marks the end of if.
If flowchart:
Example:
Idle mode
>>> x=22
>>> if x>=18 : #True
print("Ready to Vote")
Ready to Vote
Note that in idle mode after pressing enter after the print statement , nothing happens. You need to press enter again in order to run the code. This is because if is a multi line statement. But in Script mode we do not need to do this
Script Mode
x=22
if x>=18:
print ("Ready to Vote")
When we run the program, we obtain the following output :
Ready to Vote
Consider the following :
x=15
if x>=18:
print("Ready to Vote")
The above code will not give any output as the condition evaluates to false and there is no statement following the if block.
More examples
a=4
if a>0:
print ("Positive)
print ("out of if")
Output:
Positive
Out of if
In the above example a>0 is the test expression. It evaluates to true, so the statement print(:positive") is executed. The next line is print("Out of if") is also executed as the body of if ends here.
Now consider this code:
a=-2
if a>0:
print ("Positive)
print ("out of if")
Output:
Out of if
In the second code snippet, the test expression evaluates to false, therefore the statement print("Positive") is not executed. However the next statement is executed as it is not part of if.
Indentation and blocks in Python
At times it is required to execute more than one statement if the test expression evaluates to true. We call this a block of statements. To ensure this all the statements in the block should be indented with the same number of spaces. It is usually 4 spaces.
x=45
if x>20 :
print("This is a program")
print("Use Editor")
Output:
This is a program
Use Editor
Note that both the print statements are on the same indent level. Thus they are considered as a single block of statements which have to be executed when the test expression evaluates to true.
The if......else statement
The if…else statement is a variation of the simple if.. in that it provides an alternative if condition evaluates to false. If the test expression evaluates to false the statement(s) following the else are executed.
Definition
The if.. else statement executes the statement(s) given in the if block if the test expression/condition evaluates to true otherwise it executes the statement(s) in the else block.
SYNTAX
if test Expression:
Statement 1; #if block
else:
Statement 2; #else block
If the conditional expression in the parenthesis evaluates to true, statement 1 is executed otherwise statement 2 is executed.
Flowchart:
Example
x=8
if x>0:
print(x)
else:
print(x+2)
Output:
8
Example:
x=-8
if x>0:
print(x)
else:
print(x+2)
Output:
-6
In this case the output will be -6 since the test expression evaluates to false. (x+2= -8+2=-6)
Sample Program: To input a number and check whether it is negative or positive
The if......elif Statement
Python also provides a branching structure called the if....... elif statement which is used when the program requires to check for multiple expressions. There are a number of test expressions followed by statements.
Syntax
if test expression_1:
statement(s)
elif test expression_2:
statement(s)
elif test expression_3:
statement(s)
elif test expression_4:
statement(s)
.....
.....
else:
statement(s)
Explanation:
The first test expression_1 is evaluated , if it is true, the statement following the if is executed, if it is false, the test expression_2 following the elif is evaluated. If test expression_2 is true, the statement following it is executed, if it is false, the next elif test expression is evaluated and so on. Once a test expression evaluates to true, the statement following it is executed and the control comes out of the elif statement. So in effect only one of the statement or set of statements is executed.
Example
city="Delhi"
if city=="London":
print ("England")
elif city=="Sydney":
print ("Australia")
elif city=="Delhi"
print("India")
else:
print("Not Selected")
Output
India
In the above code the print statement following the matching elif ie, 'Delhi' is executed and thus the output will be India.
Sample program: Input the salary of an employee and display the Grade accordingly
Iterative Control Structures
If it is required to execute a statement or statements repeatedly or a particular number of times in a program, we use iterative control structures(loops).
An iterative control structure repeats the execution of a statement(s) for a given number of times depending on whether a given test expression evaluates to true or false.
Every loop has a loop control variable and three expressions involving it:
-
The initialization expression initializes the loop variable with some legal value.
-
The test expression determines whether or not the loop is to be continued. It is actually the condition that is checked and depending upon its value (true or false) the loop is executed or terminated.
-
The update expression is used to change the value of the loop variable for further iteration.
while loop (For Computer Science only)
The syntax of the while loop is :
while test-expression:
statement(s)
The test expression or condition following while can be any valid expression including a variable ,value or the result of a comparison. This condition determines the number of times the loop executes.
An example:
n=1 # initialization expression
while n<=4: # test expression
print (n)
n+=1 # update expression
In the above example, n=1 is the initialization expression which assigns the value 1 to the loop control variable. In the next line the while loop begins. The test expression n<=4 is evaluated. Since it is true, the body of the loop is executed which in this case contains two statements:
print(n) - prints the value of n
n+=1 - This is the update expression which increases the value of n by 1.
After the update, control goes back to the beginning of the loop where the test expression is again evaluated. If it is true the loop is executed and if it is false the control comes out of the loop. The loop is executed until the test expression evaluates to false. So the above loop will produce the following output:
1
2
3
4
Some valid test expressions:
Test-expression Explanation
x>=9 value of x is more than or equal to 9
age>20 and exp<4 age is more than 20 and experience is less than 4
The loop iterates till the test expression returns true. It breaks when the expression becomes false.
More examples
Example code
x=0
while x:
print("Loop In")
print("Loop Out")
Output:
Loop Out
Example code
p,q=2,6
while p<q:
print ("Number is",p*q)
p+=1
print (p,q)
Output:
number is 12
number is 18
number is 24
number is 30
6 6
Sample program: Print the first n integers, where n is input by the user
The for......in loop
The for loop is another iterative control structure. The for loop executes over a sequence of values specified by either a string, list, tuple or dictionary or we can use the range function. We shall study the for loop with lists, tuples and dictionary in later chapters.
The syntax :
for variable in sequence
statement(s)
where
-
variable is the loop control variable which takes a value in the sequence/list/string
-
sequence specifies is a list of values through which the loop control variable iterates
Example :
for i in "Good":
print(i)
Output:
G
o
o
d
Example:
#nums is a list here
nums=[2,5,7,9]
for x in nums:
print(x)
Output:
2
5
7
9
The range() function
The range() function generates a sequence of numbers starting from 0 by default and ending at one number before end. So the statement :
range(7)
will generate numbers from 0 through 6(7-1).
In general range(n) returns numbers 0 till n-1. We can also specify the beginning and ending values in a range. For example , range(1,5) will generate numbers from 1 to 4 in sequence.
Using the range() function
The range() function is used to specify the sequence of numbers through which a for loop control variable iterates.
Example:
for a in range(6):
print(a)
Output:
0
1
2
3
4
5
Example:
for x in range(2,6):
print(x)
Output:
2
3
4
5
We can also specify an interval between two values in a range. This is done using the optional step argument.
The general syntax of the range function is:
range( start, stop, step)
where
-
start specifies the beginning of the sequence. It is 0 by default i.e. if we do not mention explicitly
-
stop specifies where to stop. If n is the stop value, numbers till n-1 will be generated
-
step specifies an integer by which the start value increments/decrements. It is the update statement. Default value is 1
Given below is a table with examples of range function and the values generated
Example Program : Program to find sum and average of numbers between 1 and n.
Try Yourself:
Write a program to print the even numbers between -2 and -20.
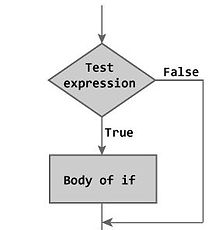
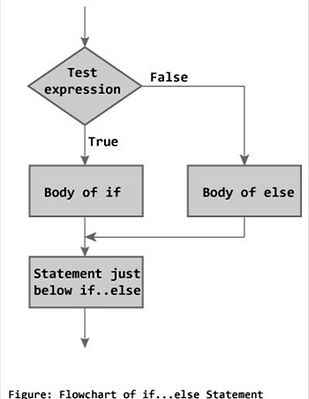
In this example , the value of x is 0. Thus the test expression while x: evaluates to false and the loop body is not executed. The control goes to the statement outside the loop which prints the message "Loop Out"
The test expression compares the two variables, p and q. Since the condition evaluates to true, the print statement is executed followed by the update expression, p+=1. The loop continues to execute till the value of p becomes 6, in which case the test expression, p<q evaluates to false. The control comes out of the loop and the print statement is executed which displays the final value of p and q.
In this example, i, the loop control variable will assume the value of the first letter in the sequence "Good" which is G. The print statement in the loop will be executed which will print G. Then the next letter 'o' will be assigned to i and printed and so on till the last value in the sequence is printed.
The range function generates the value 0 which is assigned to a. The variable a is then printed. Now a assumes the next value in the range, which is 1, and is printed. This continues till the range is exhausted. Here the start value of range is 0(by default) and the end value is 5 (6-1)
The start value of the range is 2 and end value is 5. So the sequence; 2 to 5 is printed
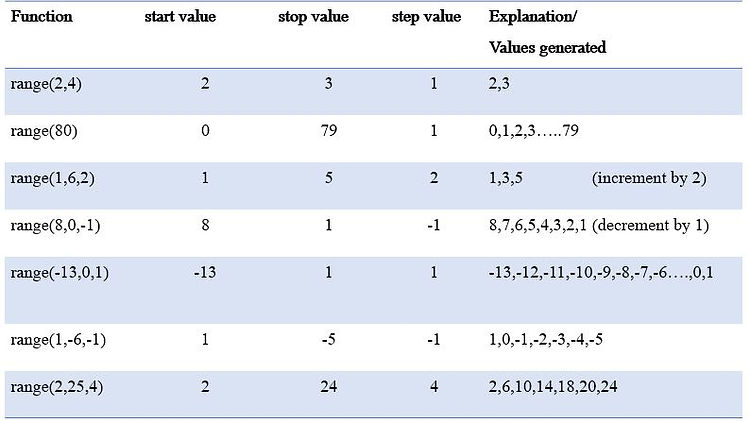