Strings in Python
Strings can be defined as a collection or sequence of character data enclosed in quotes. The quotes can enclose numbers, characters, symbols etc.. We have seen string literals in earlier chapters. Now lets take a look at how strings work in Python
Declaring a string
We can declare a string by simply assigning a variable to a string literal
str="PyPlot"
This statement declares a string named PyPlot. It is stored in the memory in contiguous locations. The figure below shows the memory representation of a string:
A string is stored as an array with index values. In python a string can be indexed in both directions. So for a string with n characters the indexing would be like:
0,1,2,3,4,.....n-1 -positive indexing
-n,-n-1,.....-4,-3,-2,-1 -negative indexing
To print a character we can refer to it using the index value. The format is:
string-variable[index value]
Example:
>>> str="PyPlot"
>>> print(str[2])
P
>>> print(str[-4])
P
>>> print(str[-2])
o
>>> print(str[5])
t
>>>
In the above example the statement print(str[2]) and print(str[-4]) will both print 'P'.
Traversing a String
We can use the for loop to traverse a string.
Example:
str1="Community"
for i in str1:
print(i)
Output:
C
o
m
m
u
n
i
t
y
Operations on Strings
There are a number of specific operations which can be carried out on strings. Lets discuss each in detail.
Concatenating Strings
The + operator can be used to concatenates strings. When applied to string literals or variables, it returns the strings joined together.
Example 1:
>>> str1="Hello"
>>> str2="Python"
>>> print(str1+str2)
HelloPython
Note that there is no space between Hello and Python.
Example 2: To add space in between the words we can concatenate a space bar enclosed in quotes:
>>> str1="Hello"
>>> str2="Python"
>>> print(str1+" " +str2)
Hello Python
Example 3:
>>> str1="Hello"
>>> str2="Python"
>>>str3="Interesting"
>>> print(str1+" "+str2+" is "+str3)
Hello Python is Interesting
Example 4:
>>> str="Rollno"
>>> print(str+2)
Traceback (most recent call last):
File "<pyshell#3>", line 1, in <module>
print(str+2)
TypeError: must be str, not int
This is a very common mistake made by programmers.
Replicating Strings using the * operator
Strings can be replicated using the * operator.
Example :
>>> str="Well"
>>> str*2
'WellWell'
Sub strings in strings: Membership Operators
There are two operators in Python which check whether a given sub string is a part of the string or not. These are 'in' and 'not in'.
The 'in' operator returns true if the sub string is a part of the string and false if not.
The 'not in' operator returns true if the sub string is NOT a part of the string and false if it is,
Examples:
>>> str="Python Pandas"
>>> "on" in str
True
>>> "pan" in str
False
>>> "Pandas" in str
True
>>> " " in str
True
>>> "o" in str
True
>>> "Py" not in str
False
>>> "h" not in str
False
>>> "B" not in str
True
>>> "daas" not in str
True
String Slicing
String slicing in Python refers to the process of extracting a sub string from a string. We can obtain a string of characters specified by two index values.
Thus for a string str, the expression str[m:n] returns a portion of the string starting at index m and upto n-1.
Example
Consider the string , “PERFORMANCE” ,:
>>> str="PERFORMANCE"
>>> str[3:6]
'FOR'
Here, m=3 and n=6
The expression str[3:6] will display all the letters starting from index value 3 up till index value 5(6-1).
Similarly, str[0:7] will give the value ”PERFORM”.
>>> str[0:7]
'PERFORM'
here, m=0 and n=7
We can also use negative indices to slice a string.
Example :
>>> str[-9:-2]
'RFORMAN'
Variations in slicing
If the first index is omitted the slice starts at the beginning that is the first character. Therefore, str[0:n] is equivalent to str[ :n].
Example:
>>> str[:7]
'PERFORM'
If the second index is omitted the slice starts at m the beginning index and continues till the end of the string.
Example:
>>> str[4:]
'ORMANCE'
Also we can use indices to complement two parts of a string. This means that for a string, str, of length n and any integer x which is more than 0 but less than n(0<=x<=n), str[:x+str[x:]=str.
Example:
str="Python strings"
I>>> str[:4]+str[4:]
'Python strings'
>>> str[:5]+str[5:]
'Python strings'
Strides in String slices
A string can also be sliced in steps, i.e, we can specify the interval between two indices. This is done by adding a third index followed by a : in the expression. This is known as a stride or step.
Syntax:
string[beg:end:step]
Example:
>>> str="Macedonia"
>>> str[1:8:2]
'aeoi'
The slicing will start from index value 1, i.e the letter 'a'. Since the step is 2, it will skip the adjacent character and the next letter will be 'e'. Similarly , 'o' and 'i' will be chosen. Thus the slice will finally be 'aeoi'.
We can also use negative values for STEP. In this case the beginning index value will be larger than the ending index value.
Example:
>>>str="Python Pandas"
>>>str[11:2:-2]
'anPnh'
Comparing Strings
Strings can be compared using the relational operators (<,>,<=, >=, ==,!=)
These operators are applied to strings in a lexicographical order(according to the dictionary)
Example:
>>> str1="Good"
>>> str2="Goat"
>>> str1<str2
False
>>> str1!=str2
True
The strings "Good" and "Goat" are compared character by character.the third letter is different in the strings. But since the ASCII code of 'o' is greater than 'a' , therefore str1 is larger than str2.
More examples:
Making changes to Strings
This is not possible as strings are immutable in Python. This means we cannot change any part of the string or modify it.
Example:
>>> str="PyhtonPAndas"
>>> str[6]='t'
Traceback (most recent call last):
File "<pyshell#25>", line 1, in <module>
str[6]='t'
TypeError: 'str' object does not support item assignment
Built in String methods
Python has a large array of in built string methods. The list is exhaustive so we shall discuss here a few of these.
The general syntax to invoke these functions is
string-object.function()
Case Conversion
1. capitalize()
This function capitalizes the first letter of the string while all other letters are converted to lowercase.
Example:
>>> str="this is a Programming Language"
>>> str.capitalize()
'This is a programming language'
2. lower()
Returns the string converted to lowercase.
Example:
>>> str="PLEAse REMOVE the COVER before OPENinG"
>>> str.lower()
'please remove the cover before opening'
3. upper()
Returns the string converted to uppercase.
Example:
>>> str="This is #YEAR 2019"
>>> str.upper()
'THIS IS #YEAR 2019'
4. swapcase()
Converts the uppercase letters to lowercase and vice versa
Example:
>>> str="GREAT this is Awesome!!"
>>> str.swapcase()
'great THIS IS aWESOME!!'
Note: The non character literals are left as it is, i.e they do not change when we apply these methods.
5. title()
Converts a string to title case by capitalizing the first character of each word in a string.
Example:
>>> str="this is Great News"
>>> str.title()
'This Is Great News'
>>>
Searching and Replacing Strings
1. replace()
This function replaces a part of the old string with a new string.
Syntax :
string.replace(old string/substring, new string/substring)
Example:
>>> str="Hello Friends"
>>> str.replace("Hello", "Good")
'Good Friends'
Note: The replace function does not make any changes to the string. The changes are only displayed.
>>> str="Hello Friends"
>>> str.replace("Hello", "Good")
'Good Friends'
>>> print(str)
'Hello Friends'
>>>
2. count()
This method counts the number of occurrences of a sub string in a string.
Syntax:
string.count(sub string)
Example:
>>> str="This is Study zone"
>>> str.count("is")
2
We can also use slicing to specify the beginning and ending of the string to restrict the search for the substring within the main string.
Example:
>>> str="Python programming on windows"
>>> str.count("on", 6,15)
0 #prints 0 as the substring "on" does not occur in the specified start and end positions
>>> str.count("on",6,25)
1 #prints 1 as the substring occurs only once between 6 and 25
3. find()
This function/method checks whether a sub string is present in a string. If the sub string is found, the index value of the first letter of the substring is returned otherwise the value -1 is returned.
Syntax:
string.find(substring)
Example:
>>> str="Casablanca"
>>> str.find("sabl")
2
The above command returns the starting index value of the substring, "sabl".
We can also restrict the search by specifying start and end values in the main string.
>> str.find("a",1,5)
1 #returns the index value of the first occurence of "a" within the string
>>> str.find("Ca",0)
0
If the sub string is not present in the string, -1 is returned.
Example:
>>> str.find("Ca",4,7)
-1 #prints -1 as the substring "Ca" is not present in the string
4. index()
This functions works exactly like the find() function except it invokes an exception or error when the sub string is not present in the main string.
Syntax:
string.index(substring)
Example:
>>> str="Casablanca"
>>> str.index("sabl")
2
>>> str.index("ball")
Traceback (most recent call last):
File "<pyshell#7>", line 1, in <module>
str.index("ball")
ValueError: substring not found
5. split()
The function splits a string into substrings. The function contains optional arguments, sep and maxsplit.
Syntax:
string.split(sep=None, maxsplit=-1)
sep specifies the character which is used to identify where to split the strings and maxsplit specifies the maximum number of splits to be applied to the string.
Example:
>>> str="we, are, good, people"
>>> str.split(sep=",")
['we', ' are', ' good', ' people']
>>> str="This . is. real.cool"
>>> str.split(sep=".")
['This ', ' is', ' real', 'cool']
6. partition()
This method searches for the string specified in the brackets and then returns a tuple having three elements. The first is the part before the string, the second is the specified string and the third is the part after the string.
Syntax:
string.partition(specified string/string variable)
Example:
>>>str="Good morning everyone. Welcome to the course"
>>>print(str.partition("Welcome"))
('Good morning everyone. ', 'Welcome', ' to the course')
7. strip()
This method returns the string after removing both the leading and trailing spaces. There is an optional parameter chars, which can be used to specify the a set of characters to be removed from left or right of the string.
Syntax:
string.strip([chars])
Example(without using char):
str=" Good morning "
print(str) # without the strip method, str is printed with leading and trailing spaces
print(str.strip())
Output:
Good morning
Good morning
Example(using char):
str=" odshoo omoo do tod "
print(str.strip(' od ')) # removes the characters 'od' from the left and right of the string along
#with spaces
Output:
shoo omoo do t
8. lstrip()
It returns a copy of the string with the leading spaces(left) removed.
Syntax:
string.lstrip([char])
This function also has an optional parameter char which removes specified characters from the left along with the spaces .
Example:
str=" odshoo omoo do tod "
print(str.lstrip()) # removes leading spaces only
print(str.lstrip(' od ')) # removes the characters 'od' along with leading spaces
Output:
odshoo omoo do tod
shoo omoo do tod
9. rstrip()
It returns a copy of the string with the trailing spaces(right) removed.
Syntax:
string.rstrip([char])
This function also has an optional parameter char which removes specified characters from the right along with the spaces.
Example:
str=" odshoo omoo do tod "
print(str.rstrip()) # removes trailing spaces only
print(str.rstrip(' od ')) # removes the characters 'od' along with trailing spaces
Output:
odshoo omoo do tod
odshoo omoo do t
Character Classification functions
These functions classify a string depending on the type of characters it contains.
1. isalnum()
This checks whether the target string consists of alphanumeric characters. It evaluates to true in this case otherwise returns false.
Example:
>>>str="Xyz234"
>>>str.isalnum()
True
>>>str="ABC##90"
>>>str.isalnum()
False #contains special characters "##"
>>>str=" "
>>>str.isalnum()
False #str is an empty string
2. isalpha()
This function checks whether the string consists of alphabets. It returns true if yes and false if it contains any other character(other than an alphabet)
Example:
>>>str="Collection"
>>>str.isalpha()
True
>>>str="Collection18"
False
3. isdigit()
Checks whether the given string contains of digits. It returns true if all the characters in the string are digits, false otherwise.
Example:
>>>str="9923"
>>>str.isdigit()
True
>>>str="9934B"
>>>str.isdigit()
False
4. istitle()
Checks whether the string is in title case. If only the first letter(alphabet) of every word in the string is in uppercase and the rest are in lowercase, it returns True otherwise it returns False.
Example:
>>>str="Return the Text book"
>>>str.istitile()
False
>>>"Good Work Is Exemplary".istitle()
True
5. isupper()
Checks whether each letter of a string is in uppercase. If all the letters are in uppercase, it returns True, False otherwise.
Example:
>>>str="POLLEN"
>>>str.isupper()
True
>>>str=FallEn"
>>>str.isupper()
False
6. islower()
Determines whether the characters of the string are in lowercase. It returns True if every character/letter of the string is in lowercase, False otherwise.
Example:
>>>str="decathlon"
>>> str.islower()
True
>>> 'The great urban place'.islower()
False
7. isspace()
Check if all the characters in the text (string) are whitespaces:
Example:
>>> str="Good Morning"
>>> str.isspace()
False
>>> str=" "
>>> str.isspace()
True
Built in functions
These are similar to methods. They are invoked without using the object in the call statement
1. chr()
This function converts an integer to its equivalent ASCII character.
Example:
>>> chr(9)
'\t'
>>> chr(65)
'A'
>>> chr(98)
'b'
>>> chr(110)
'n'
>>> chr(22)
'\x16'
>>> chr(40)
'('
2. len()
It returns the length of the string.
Example:
>>> str="Hello Patrick"
>>> len(str)
13
Note: Spaces in a string are counted as the length of the string.
3. ord()
This function returns the numeric value represented by a character. It converts the character to its equivalent numeric value.
Example:
>>>ord('a')
97
>>>ord('"')
34
Some specific Functions
1.startswith()
This function returns True if the string starts with a specified value, otherwise False.
Syntax
string.startswith(value, start, end)
Here:
value is the value that is being checked with which the string starts (for in the given
string, string)
start is an optional integer parameter which when used specifies the position to start
the search
end is an optional integer parameter which when used specifies the position to end
the search
Example:
>>> str="Education is the key to Success"
>>> str.startswith("Education")
True
Another Example:
>>>str="Education is the key to Success"
>>>str.startswith("Education", 1,9)
False
>>>str.startswith("Education", 0,9)
True
2. endswith()
This function returns True if the string ends with a specified value, otherwise False.
Syntax:
string.endswith(value, start, end)
Here:
value is the value that is being checked with which the string ends( for in the given string, string )
start is an optional integer parameter which when used specifies the position to start
the search
end is an optional integer parameter which when used specifies the position to end
the search
Example:
>>>str="Precious stones are rare"
>>>str.endswith("rare")
True
>>>str="Precious stones are rare"
>>>str.endswith("rare", 2)
False
>>>str="Precious stones are rare "
>>>str.endswith("rare")
False # Since the string has spaces after the word 'rare', it will not match
>>>str="Precious stones are rare"
>>>str.endswith("rare", -4)
True #Here the start is a negative number specifying to start from the end of the string
Sample program: Write a program to check if a string is a palindrome.
A palindrome is a String which when read from either side gives the same output. eg, MadaM, level, radar, noon etc.
Method 1:
Explanation
1. Take two indices, one from beginning of string, one from end; i and j
2. Set a Boolean variable flag to True which assumes string to be palindrome
3. Run loop till half of the string is traversed
4. Compare first element and last element, then second and second last and so on till we reach halg of the string
5. If even one character does not match, set flag to False
6. Display appropriate message depending on value of flag
Method 2:
We cannot concatenate a string and a numeric operand. Both the operands have to be strings otherwise an error will be generated
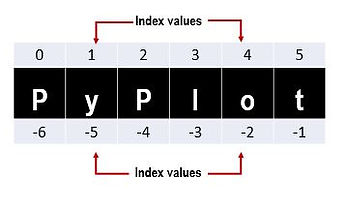
Python is case sensitive, so this returns false
Checks for a single space in the string

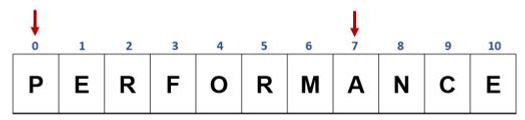