Python Basics
Like every language, Python has some basic building blocks, called tokens. These are
-
Python character set
-
Keywords
-
Comments
-
Constants
-
Operators
-
Variables
-
Data Types
Let us discuss each one of these in detail. Operators, variables and data types are covered in detail in the website.
Character Set :
A character set of a language is the range of permissible characters that can be used in a program written in that language. The Python language includes the following character set :
All the ASCII letters, ie. A-Z,a-z, 0-9, special characters like +,-,#,%, *,/,//,% and many more.
Keywords
A keyword is a word that has some predefined meaning in the language. These are also known as reserved words. Some characteristics of keywords:
-
Keywords(Reserved words) are written in lowercase except a few in sentence case(False, None and True).
-
We cannot use a keyword as variable name, function name or any other identifier.
The following is the list of some common keywords in Python:
Keywords can be altered in different versions of Python. To view the complete list of keywords, we can use the following code:
>>> import keyword
>>> print(keyword.kwlist)
Comments
In programming languages, comments are used to describe or explain the purpose of the code. We can compare it to labeling a diagram in science. Comments are not executed and are completely ignored by compilers and interpreters.
In Python any text written to the right of a # is a comment.
For example:
>>>print ("Hello") #This is a simple print statement
Constants
A constant or a literal is a value that does not change. Constants can be numbers, fractions or words or letters. For example, 2 is a number constant, 20.5 is a fractional constant , "Hello and welcome to Python" is a word(string of characters) constant. These are values assigned to variables in Python.
Escape Sequences: These are special constants which have a functionality attached to them. We can use them mostly with the print statement to give variation in output. Listed below are some escape sequences with their explanation.
Operators
These are those lexical units that trigger some computation when applied to variables and other objects in an expression. Operators require some data to operate on and such data is called operands. For example
Consider the expression
>>>4+5
9
Here 4 and 5 are the operands and + is the operator. Python provides us with a number of operators of various types. We shall cover operators in detail later.
Variables
A variable in Python is a storage location in the memory which is used to store data. The data value can be either assigned to the variable or input by the user.
Also as the name suggests the value stored in a variable can change.
Data Types
The computer cannot differentiate between the value 2 and "A". For the computer both are simple data values. So we need to specify the type of data to store using data types. When we define variables ,we need to specify their data type.Data types specify the type of value a variable can have and the set of permissible operations that can be performed on it.
Python supports various data types which are covered in detail.
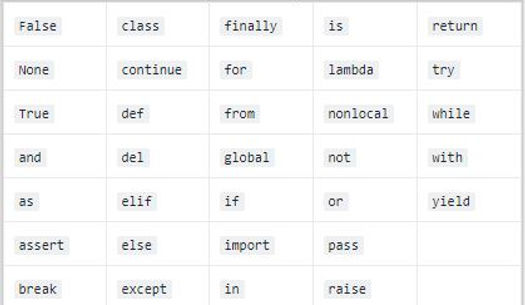
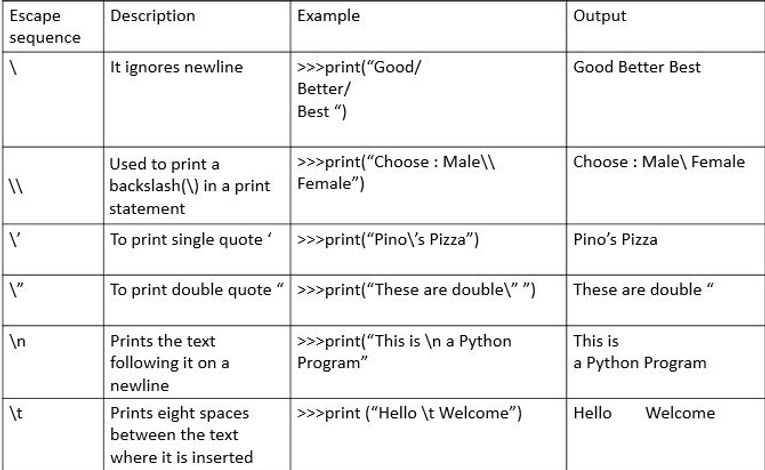
Python is a case sensitive language. This means that words written in different cases are treated differently.