Lists in Python
Table of Contents
Accessing lists using Index value
List Operations
Finding maximum and minimum values
List Comprehension using for loop
marks=[56,78,87,90,78,90,87,90,45,89,56,90,65,79,97,98] #List of given marks
number=int(input("Enter the marks")) #marks to be input
freq=0 #counter set to 0
for i in marks:
if i==number:
freq=freq+1 #for each occurrence of the number increment counter by 1
print("The frequency of marks", number , " is:", freq)
Output:
Enter the marks 90
The frequency of marks 90 is: 4
A list is a data type in Python defined by comma separated objects enclosed in square brackets. Examples of Lists are :
a=[2,4,6,8]
Type=['PAN', 'LAN', 'MAN', 'WAN']
x=[]
marks=[65.5,78.9,56.4,79.5, 96.5,60.0]
Characteristics of Python Lists
-
Lists are ordered
-
Lists can contain any type of objects
-
The elements can be accessed using index values
-
They are mutable
-
Lists are dynamic
-
Can be nested
A python list is an ordered list of objects
This means that when we define a list, the order in which we specify the elements remains the same for the scope of the list. For example:
>>> names=['Lucy', 'John', 'Peter', 'Mary']
>>> names2=['John','Peter','Lucy', 'Mary']
>>> names==names2
False
>>> n=['Lucy', 'John', 'Peter', 'Mary']
>>> n==names
True
The order of list names and names2 do not match therefore they are not equal and comparison gives a False. On the other hand the order in list n is the same as in names thus comparison generates a True.
Lists support heterogeneous elements
This means lists can contain objects or elements of the same type or of different types.
Example
>>> a=[20,40,60,80.90,56]
>>> b=['cat', 'bat','mat','rat']
a contains a list of integers whereas b contains a list of strings. But a list can also contain values of different types .
Example:
>>> x=[1,'cat',45.6,'A']
>>> print(x)
[1, 'cat', 45.6, 'A']
>>>y=[22,'garden',True, 90.9]
The items or elements in a list may be repeated. Also the list can contain any number of elements or objects.
Lists can be accessed by Index Values
Just like Strings, lists can be accessed using index values. The index values can be positive(left to right) or negative( right to left).
list-variable[index value]
Example:
>>>no=[10,20,30,40,50,60,70]
Memory representation:
>>>no[4]
50
>>>no[-2]
60
Similarly for a list of strings
>>>a=['cat', 'bat','mat','rat']
Memory Representation:
>>>a[1]
'Bat'
>>>a[-4]
'Cat'
List Operations
Slicing
Just like strings, lists can also be sliced. We can use positive as well as negative indices. The syntax for slicing a list is :
list-variable[starting index value(default 0):ending index value(element n-1 ): stride/step value(default 1)]
Example:
>>> a=[10,20,30,40,50,60]
>>> a[2:4]
[30, 40]
>>> a[:4]
[10, 20, 30, 40]
>>> a[2:]
[30, 40, 50, 60]
>>> a[-6:-1]
[10, 20, 30, 40, 50]
We can also use strides or steps to access the list elements in a particular sequence.
>>> a=['Cat', 'Bat','Mat', 'Rat']
>>> a[0:4:2]
['Cat', 'Mat']
The code above; a[0:4:2] will display the list items starting from index value 0 till value 3[4-1]. The number 2 indicates the step or stride which means that items will be displayed after skipping the adjacent value. Therefore the values of a[0] and a[2] will be displayed as indicated below.
We can have negative steps as well.
Example
>>> a=[10,20,30,40,50,60]
>>>a[5:2:-2]
[60, 40]
In this case the starting index has to be larger than the ending index.
In the example below an empty list is returned as the starting index is less than the ending index and the step is negative.
>>> a[1:4:-1]
[]
A list can be reversed by using the slicing operation with empty starting and ending index values and a step with the value -1.
>>> a=[10,20,30,40,50,60]
>>> a[ : : -1]
[60, 50, 40, 30, 20, 10]
Lists are Mutable
Lists are one of the few data types which are mutable in Python. Unlike strings, we can change the elements of a list.
>>>a=[20,25,30,35,40,45]
>>>a[2]=38
>>>a
[20,25,38,40,45]
In the above example we are modifying a single list element. But we can modify multile elements as can be seen in the following sections.
Modifying lists using slicing
Slicing can be used to overwrite the elements of an existing list and thus modify it.
Example:
>>> a=[10,20,30,40,50,60]
>>> a[0:2]=['Cat','Bat'] #Replacing elements 0 to 1 in the list a, thus 10, 20 will be replaced
>>> a
['Cat', 'Bat', 30, 40, 50, 60] #Modified List
Example:
>>> a=[10,20,30,40,50,60]
>>> a[2:6]=['j', 'k', 'l', 'm', 'n'] #Replacing elements 2 to 5
>>> a
[10, 20, 'j', 'k', 'l', 'm', 'n'] #Modified List
Consider the following statements.
>>> x=[1,2]
>>> x[2:]="456"
>>> x
[1, 2, '4', '5', '6']
The above list, x, is being appended by the sequence "456". Thus '4','5' and '6' are added to the list after the value 2. Also , x[2:] specifies the starting position where the new sequence is to be placed.
But when we try to add a number as below:
>>> x[4:]=789
Traceback (most recent call last):
File "<pyshell#6>", line 1, in <module>
x[4:]=789
TypeError: can only assign an iterable
This happens because 789 is a number not a sequence. On the other hand if we use indexes way out of range, the sequence is just appended at the end of the list as a sequence.
>>> x=[2,3,4,5,6,7,8,9]
>>> x[20:]="1122"
>>> x
[2, 3, 4, 5, 6, 7, 8, 9, '1', '1', '2', '2']
Traversing a List
Traversing means visiting each element of the list. We can traverse a Python list using loops.
Example:
a=[20,40,50,60]
for i in a:
print(i)
Output:
20
40
50
60
Example:
a=["Cat","Bat","Rat","Mat"]
for i in a:
print(i)
Output:
Cat
Bat
Rat
Mat
Sample Program : In a list of numbers multiply all even elements by 2 and all odd elements by 3
a=[20,45,43,78,90,24]
for i in a:
if i%2==0:
i=i*2
else:
i=i*3
print(i)
Sample Output:
40
135
129
156
180
48
Sample Program : Search for a given word in a list:
a=["Cat","Bat","Mat","Rat"]
found=False
search=input("Enter a word to be searched :")
for i in a:
if i==search:
print("Found")
found=True
if found==False:
print("Not Found")
Sample runs and Output:
Enter a word to be searched : Mat
Found
Enter a word to be searched : ball
Not Found
Try yourself :Program to search for a given word(input by the user )in a list .
Joining lists using the '+' operator.
Lists can be joined by using the concatenation operator,'+'.
>>> L1=[2,4,6,8]
>>> L2=[1,3,5,7]
>>> L1+L2
[2, 4, 6, 8, 1, 3, 5, 7]
Lists need not be stored in a variable to be concatenated. We can also join list literals using the '+' operator.
>>> ['Bat','Rat']+['Pat','Cat']
['Bat', 'Rat', 'Pat', 'Cat']
>>> [22.5,89]+['pot','bot']
[22.5, 89, 'pot', 'bot']
>>> [22.5,89]+['pot','bot']+[False,90]
[22.5, 89, 'pot', 'bot', False, 90]
Replicating lists using the '*' operator.
The '*' operator can be used to replicate lists.
>>>L1=[2,4,6,8]
>>> L1*2 #Multiplying the list by 2
[2, 4, 6, 8, 2, 4, 6, 8] #The list is displayed twice
>>> ['Bat','Rat']*4
['Bat', 'Rat', 'Bat', 'Rat', 'Bat', 'Rat', 'Bat', 'Rat']
Using other Relational operators with lists.
We can use all the relational operators with lists. Given below are the sample code and outputs.
>>> [1,2,3,4]>[2,3,4,5] #the elements of list on the left are smaller than the one on right
False
>>> ['cat','bat','mat','rat']==['bat','mat','rat','cat']
False #Even if both the list contain same words, the placement matters
>>> ['cat','bat','mat','rat']>['bat','mat','rat','cat']
True
>>> [False, True]!=[True, False]
True
To be able to compare two lists using relational operators, the elements in the list must be of the same datat type..
>>> [13,'cat',60.5,22]>['bat',22,34.5,2]
Traceback (most recent call last):
File "<pyshell#3>", line 1, in <module>
[13,'cat',60.5,22]>['bat',22,34.5,2]
TypeError: '>' not supported between instances of 'int' and 'str'
Built in functions
There are a number of built in functions which can be used with lists. Let us discuss each one in detail.
1. append()
Syntax:
list. append(object)
object can be a number, string or another list, dictionary etc.
The function append() is used to add a single item at the end of the list.
>>>x=[1,2,3,4]
>>>x.append(7)
>>> print(x)
[1, 2, 3, 4, 7]
The element is added at the end of the list by modifying it, no new list is created. Strings can be appended in a similar way as a single entity.
>>> a=['cat','mat','rat']
>>> a.append('bat')
>>> a
['cat', 'mat', 'rat', 'bat']
The append function adds the argument at the end of the existing list as a single entity. This means if we try to append a list using this method, the list will be added at the end as a single element.
For example:
>>> a=[1,2,3]
>>> a.append([6,7,8]) # appending a list of numbers
>>> a
[1, 2, 3, [6, 7, 8]] # The list is appended as a single item
When we try to append more than one item as separate entities, we get an error.
>>> a.append(6,7,8)
Traceback (most recent call last):
File "<pyshell#3>", line 1, in <module>
a.append(6,7,8)
TypeError: append() takes exactly one argument (3 given)
2. extend()
Syntax:
list.extend(iterable)
Iterable can be another list, dictionary etc.
The extend() method can be used to concatenate two lists. The second list is added at the end of the first list.
>>> m=[1,2,3]
>>> m.extend([5,6,7])
>>> m
[1, 2, 3, 5, 6, 7]
The argument in the extend() method must be an iterable, ie a list.
>>> m=[1,2,3]
>>>n=[8,9,4]
>>> m.extend(n)
>>> m
[1, 2, 3, 8, 9, 4]
3. insert()
This function inserts a new value in the list at a given postion.
Syntax:
list.insert(index, value)
index is the position in the list where the new value is to be inserted.
The rest of the items in the list are pushed to the right.
Example:
>>> a=[2,4,7]
>>> a.insert(2,90)
>>> a
[2, 4, 90, 7]
>>> a.insert(1,"Good")
>>> a
[2, 'Good', 4, 90, 7]
We can also insert an iterable , e.g. we can insert a list.
>>> a.insert(3,[2,3,4])
>>> a
[2, 'Good', 4, [2, 3, 4], 90, 7]
4. reverse()
Syntax:
list.reverse()
This function simply reverses a list, by changing the order of the elements in a list.
Example:
>>> a=[22,44,56,11,99, 88]
>>> a.reverse()
>>> a
[88, 99, 11, 56, 44, 22]
5. index()
Syntax:
list.index(item)
This function returns the index value of the first occurrence of an item in the list.
Example:
>>> city=["Delhi","Mumbai","Chennai","Kolkatta"]
>>> city.index("Mumbai")
1
If there are more than one occurrences of an item , the index value of the first occurrence only will be returned.
>>> list=[2,3,4,2,5,6]
>>> list.index(2)
0
6. sort()
Syntax:
list.sort()
This function sorts a list in an ascending order by default.
Example:
>>> a=[22,44,56,11,99, 88]
>>>a.sort()
>>> a
[11, 22, 44, 56, 88, 99]
7. len()
Syntax:
len(list)
It simply returns the length, i.e. the number of elements in a list.
Example :
>>> a=[22,44,56,11,99, 88]
>>>print(len(a))
6
8. count()
Syntax:
list.count(element)
This function returns the number of occurrences of a particular element in the list.
Example:
>>> y=[22,66,77,44,66,88,99,22]
>>> y.count(22)
2
>>> y.count(99)
1
>>> y.count(38)
0
>>> y.count(-99)
0
9. clear()
Syntax:
list.clear()
This function simply removes all elements from the list.
Example:
>>> y=[22,66,77,44,66,88,99,22]
>>>y.clear()
>>> y
[]
10. remove()
Syntax:
list.remove(object)
This function removes an object specified in the brackets, from the list.
Example:
>>> x=[2,4,5,2,7,9,8,9]
>>> x.remove(8) #to remove number 8 from the list
>>> x
[2, 4, 5, 2, 7, 9, 9]
>>> Fruits=["Apple","Mango","Guava","Orange","Papaya"]
>>> print(Fruits)
['Apple', 'Mango', 'Guava', 'Orange', 'Papaya']
>>> Fruits.remove("Guava")
>>> Fruits
['Apple', 'Mango', 'Orange', 'Papaya'] #the remove statement removes "Guava" from the list
If we try to use the remove() an object which does not exist, an error occurs.
>>> Fruits.remove("Kiwi")
Traceback (most recent call last):
File "<pyshell#50>", line 1, in <module>
Fruits.remove("Kiwi")
ValueError: list.remove(x): x not in list
11. pop()
Syntax:
list.pop(index)
The method also removes an item whose index value is mentioned from the list. It is different from the remove method in two ways; it deletes the element whose index value is mentioned , and it returns the item which is deleted.
Example(considering the list mentioned in the previous example):
>>> Fruits.pop(3)
'Papaya'
>>>Fruits
['Apple', 'Mango', 'Orange']
If we use the pop function without the index value, the last element in the list will be removed.
Example:
>>> Fruits.pop()
'Orange'
>>> Fruits
['Apple', 'Mango']
The pop() function when used with out of range index will give an error.
Example:
>>> x=[2,4,5,2,7,9,8,9]
>>> x.pop(9)
Traceback (most recent call last):
File "<pyshell#55>", line 1, in <module>
x.pop(9)
IndexError: pop index out of range
We can use negative indexing with the pop() function.
Example:
>>> x=[2,4,6,8,9,13]
>>> x.pop(-1)
13
Searching in a List
Searching is an operation fundamental to programming. It is implemented in all computer languages. The practical applications include searching for a number in a list of numbers or a name in a list of employee names. Also sometimes we need to find minimum or maximum value in a list. Programmers are required to model such real life operations.
Let us take a look at searching in a list.
Searching a value:
Suppose we need to search for a value in a list. For this we can use the in operator.
Example:
>>>listA=[250, 450. 'Country', 34.5]
>>>450 in listA
True
Example : Program to search for a given city in a list of cities.
Finding the maximum and minimum values in a list
max() and min()
The function max() returns the highest value in a list when applied whereas the function min() returns the lowest. To be able to use these functions, the list must be homogeneous, i.e. all the values in the list have to be of the same data type.
e.g.
>>> list1=[89,99,78,65,90,34]
>>> max(list1)
99
>>> min(list1)
34
Similarly:
>>> letters=['h','A','E','r']
>>> max(letters)
'r'
>>> min(letters)
'A'
With character data type the list values are compared using the ASCII code.
Sample Program : To find the number of occurrences of a given value in a list.
Sorting
Sorting is arranging the data elements in a particular order. The values in list can either be arranged in ascending or descending order.
Consider following list of integers as the marks obtained by 7 students in a test:
3, 12, 6, 8, 5, 9, 15
After sorting the list elements appear as :
3, 5, 6, 8, 9, 12, 15
There are a number of ways to sort a given list of elements. A number of algorithms have been devised to sort list of elements. Depending on the size of the list, each sorting technique offers a unique solution to sorting a list of values. In the context of this course we will be studying following sorting algorithms:
-
Bubble sort
-
Insertion sort.
Bubble Sort
In this technique, two adjacent elements in a list are compared. Depending on the required order of sorting(ascending or descending), the two numbers are swapped. If the sorting is to be done in ascending order; if first number is bigger than the first, they are swapped. Similarly in descending order if the second element is larger than the first element both are swapped. The entire list is traversed and the same technique is applied, As a result, after the first iteration(pass) of the list the largest(or smallest) element in the list id bubbled out. to the end of the list.
The entire list is traversed repetitively till all the elements are sorted.
The algorithm and code for Bubble sort is given below:
-
START
-
DECLARE TWO VARIABLES M AND N
-
INITIALIZE N=0,
-
INITIALIZE M=0
-
SET A VARIABLE L= Length of the list, L=len(list)
-
If list[M]>list[M+1] THEN SWAP VALUE OF list[M] with list[M+1]
-
SET M=M+1
-
IF (M<L-1) GOTO STEP 4
-
SET N = N +1
-
IF N<L GOTO STEP 3
-
STOP
Code:
Insertion Sort
In this type of sorting, the list is divided into two parts, the sorted and
unsorted part. The first element from the unsorted sub list is inserted into
the correct position in the sorted sub list.
This is repeated till the entire of the list is sorted.
The following example illustrates how the insertion sort algorithm works:
1. The list is unsorted in the beginning.
2. The first element, 5 is considered sorted sublist.
3. The first element in the unsorted part is 1. It is inserted before 5 , in its correct position
4.Since 5 and 8 are larger than 1 no change occurs.
5. Then the number 3 is encountered and inserted into its correct position in the list.
6. Similarly the entire list is scanned and sorted
Code :
a list of integers, having 4 elements
a list of strings, having 4 elements
an empty list
a list of decimals or floating point numbers, 6 elements

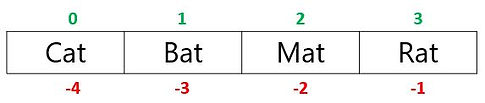
positive indices
negative Indices
positive indices
negative Indices

#Bubble Sort
list=[14,56,8,22,78,6] #list to be sorted
n=len(list)
for i in range(n) #traversing list
for j in range(0,n-1) #traversing sublist
if list[j]>list[j+1]
list[j],list[j+1]=list[j+1], list[j] #swapping
print("After Sorting", list)

#Insertion Sort
list=[24,56,7,89,90,11]
k=len(list)
for i in range(1, k):
numd = list[i]
j = i-1
while j >=0 and numd < list[j] :
list[j+1] = list[j]
j -= 1
list[j+1] = numd
List Comprehension in Python
We can also use for loops to create lists in Python using list comprehension. The general syntax for creating lists in this way is :
listvariable=[ list element(iterator) for iterator in range(n)]
Example:
list2=[i for i in range(4) ]
print(list2)
Output:
[0, 1, 2, 3]
We can also use conditions while forming lists.
Example:
list2=[i for i in range(25) if i % 2==0]
print(list2)
Output:
[0, 2, 4, 6, 8, 10, 12, 14, 16, 18, 20, 22, 24]
Using Strings, we can create lists of alphabets.
Example:
list1=[i for i in 'humane']
print(list1)
Output:
['h', 'u', 'm', 'a', 'n', 'e']
Example:
str1=input("Enter a word :")
list1=[i for i in str1 if str1.isupper()]
print(list1)
Output:(option 1)
Enter a word :GOOD MORNING
['G', 'O', 'O', 'D', ' ', 'M', 'O', 'R', 'N', 'I', 'N', 'G']
Output:(option 2)
Enter a wordGood Morning
[]